Table of Contents
Introduction
Back in 2016 my better half received the hexagon bugs puzzle as a gift. Consequently, I felt a strong urge to solve this hexagon tile puzzle, but I found it to be pretty hard. Therefore, I gave up pretty soon after I realized the grind it took. Shortly afterwards, I started thinking about how I could write an algorithm to solve it for me. The result might not be the best code I wrote, but it did solve the puzzle. I kept the code in a private repository since and decided to make it public.
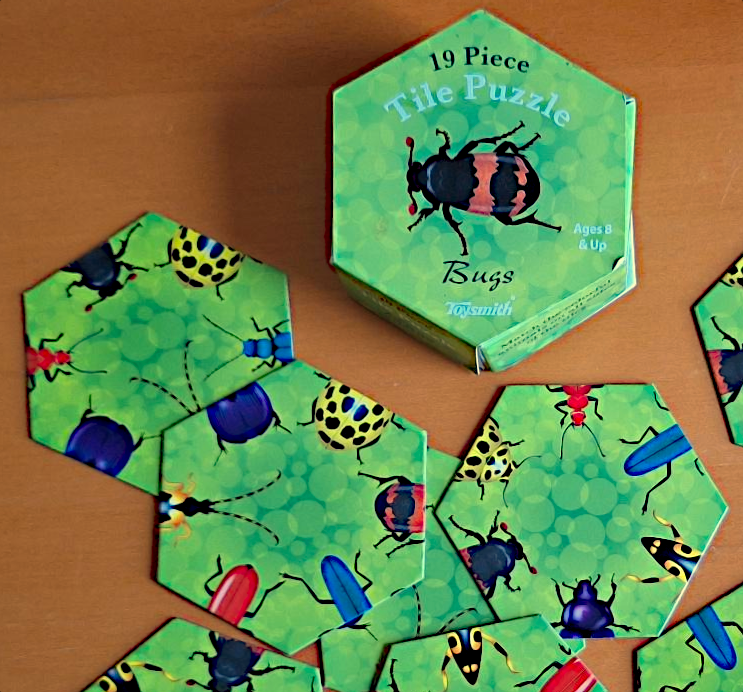
Prerequisites
I needed to do some pre-work before I was able to delve into writing the code. Therefore, I marked all cards on the back with a number. I gave every insect type a unique identifier and another identifier whether it was the back or the front of the insect. Then I gave each of the six sides of the card a mark as well, so I knew for each side what insect type there was and whether it was the front or the back on that specific side.
I set up some classes and variables to store basic information:
- An array with unplaced Cards, called the Deck. The cards are in numeric order in this array.
- A Board. A board has placeholders for each Card, I called these placeholders Tiles. The placeholders are already in the shape of the solution.
- Each Tile holds information about its adjacent tiles and the Card on it. It tracks the highest card number placed on it for a certain attempt as well.
- A Card has information of its sides, rotation and which Picture is on the side for that rotation.
The algorithm (description)
Before we can start to solve the hexagon tile puzzle, I started to think about which logical steps are needed beforehand. These steps are in order, unless a step specifically points to go to another step. If a) or b) is specified, only one of both will be true. If I write “we”, I mean the algorithm or the system with it.
Glossary
- Deck: The deck of cards that we are about to place.
- Unplaced deck: The cards that we could not match so far.
- Match: A successfully connected bug, front to back.
- Board: The shape of the solution, it consists of 19 Tiles.
- Tile: A placeholder for the end shape of the puzzle. We place a Card on each Tile.
- Card: A hexagon shaped card with on each of the six sides the front or back side of a specific bug type.
/**
* The Board, where each number is a Tile
* 05 is connected to: 01, 02, 04, 06, 09 an 10
*
* 01 02 03
* 04 05 06 07
* 08 09 10 11 12
* 13 14 15 16
* 17 18 19
*/
JavaLogic
The algorithm starts with an ordered Deck of Cards and with a deck of “unplaced” cards, which starts empty. Unplaced means that it could not connect successfully (match) to adjacent Cards. A match means the same bug type with a front connected to a back. This needs to be true for each connection of a Card.
Steps
- Start loops: For each card in the Deck and then for each rotation of that Card we go through the following:
- Place the top card from the Deck on the first empty placeholder (Tile), but only if the Card number is higher than the latest placed Card on that Tile. Now one of the following is true:
- a) If this is possible, go to step 3.
- b) If this is not possible and the Deck is not yet depleted, we try the next card in the Deck. The last tried Card goes into the Unplaced deck. The Tile receives the number of the Card that was placed on it last.
- c) If the deck is depleted: We remove the current and previous Card on the respective two Tiles. If we had to remove the very first Card on the Board, we go to additional step I and then to step 2 with the next iteration from step 1.
- d) There is a solution.
- Check if there is a match on each connected side of the Card. We go to additional step I either how.
- a) If this is possible, keep the Card on the Tile (placeholder) and then we go back to step 2.
- b) If this is not possible the algorithm rotates the card for a maximum of six times. If it cannot be matched with all adjacent Cards, the Card is placed in the “Unplaced” deck. The algorithm removes the Card from the Tile. Go to step 2.
Additional step
I) Put all cards from the “unplaced” deck into the regular Deck.
Graphical interface
In the first version of the algorithm, the text output gave me the location of each card number on the Board. Later I decided to scan all cards and added a number with Gimp on it. I then made the algorithm visible with the help of some graphical libraries (awt, Swing).
Algorithm in action
Conclusion
There are a few “problems” with the algorithm which I did not bother to solve, because I reached my goal. The algorithm did in the end solve the hexagon tile puzzle. One of the problems is that in the very first iteration it checks for a single placed card if there are matches, which clearly can’t be the case.
In the end, the compelling allure of the unsolved mystery has gracefully receded, for the solution has been found. The once insatiable thirst for resolution is now quenched, leaving behind a serene satisfaction in its wake.
The code is on GitHub, which needs (not for cloning) access tokens to access nowadays.