Explanation
The Facade design pattern is a structural pattern that provides a simpler interface to a complex subsystem. For example, envision that you imported several third-party libraries for your project and you need them all to solve a specific problem. With your facade class you can make a single interface (in a general sense) which uses all of these libraries in the background.
Smart home devices that you can all control via Google Home or Alexa are an example of the facade pattern. Voice recognition controls several devices at once and possibly in a specific way. Other practical examples would be: autopilot on a plane, a self-driving car, a climate control system, a washing machine, and so on. A few relatively simple button presses translate via the facade, which then directs one or more subsystems.
Use cases
- You want to simplify the use of one or more complex systems
- You want to create more overview in your code
- You want to create a new interface for existing objects
Pitfalls
- It can be unclear what exactly happens in the background and what systems will be used
- If something in a subsystem breaks, so will the call via the facade
- Too many coupled classes to the facade can give it too much responsibility
Example
In this example we will create an image processor. Imagine that every time that we upload an image to our website, we want to ensure that it will be compressed, resized, and converted to a specific file type. The underlying requirements would be that the image will load quickly, has a consistent file type and size compared to other uploaded images.
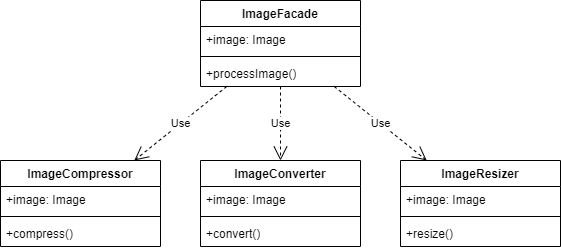
// The libraries in this example do not really exist (obviously)
using Library1.ImageCompressor;
using Library2.ImageConverter;
using Library3.ImageResizer;
namespace DesignPatterns.Facade
{
public class Facade
{
// the facade prevents you from using the library classes directly
public Image ProcessImage(Image img)
{
img = ImageCompressor.Compress();
img = ImageConverter.Convert("png");
img = ImageResizer.Resize(1024, 1024);
return img;
}
}
}
C#using DesignPatterns.Facade;
class Program
{
static void Main(string[] args)
{
Facade facade = new Facade();
Image img = new Image();
img = facade.ProcessImage(img);
}
}
C#Conclusion
The facade design pattern might be a relatively simple pattern, in contrast to the factory method design pattern for example. You can use this pattern to hide complex functionality and put it behind a simpler interface. In the example above, we automated the processing of images with the help of one single function call.
References
Freeman, E., Bates, B., & Sierra, K. (2004). Head first design patterns.
Gamma, E., Helm, R., Johnson, R., & Vlissides, J. (1994). Design Patterns: Elements of Reusable Object-Oriented Software.
Wikipedia contributors. (2024, 10 september). Software design pattern. Wikipedia. https://en.wikipedia.org/wiki/Software_design_pattern