Table of Contents
Introduction
The Chain of Responsibility is a behavioral software design pattern. The pattern is based on events and event handlers where the event goes through a chain of registered handlers. Each handler in the chain either does something with the event, or passes the event on to the next handler. The handler could also be designed to process the event and also pass it on.
Use Cases
- You want to handle events in a GUI, like mouse clicks.
- You want to log events and based on criteria choose the way of logging that fits best.
- You want to use multiple safety checks before authorizing someone.
- You want to create a chain of escalation.
Pitfalls
- You’ll have to implement feedback to know which handler handles the event.
- An event (or request) may not be handled at all.
- The inner workings of the chain and decision making are unclear.
Java Example
Let’s create an incident handler that demonstrates the pattern. We’ll have three severity levels of logging, MINOR incidents, MAJOR incidents and CRITICAL incidents. For repudiation we’ll just log a minor incident. A major incident will be automatically emailed to the administrator. In the case of a critical incident, the IT supplier will automatically get a notification. We won’t implement these three features, just the structure of the chain of responsibility design pattern.
Interface and abstract class
An incident should be able to be chained to another incident and it should be handled. We can create an Interface for these two functions.
package com.chosengambit.chain;
public interface IIncident {
void setNextHandler(AbstractIncident nextHandler);
void handleIncident(AbstractIncident.IncidentType type, String message);
}
JavaNow we can design the AbstractIncident class, which holds the functionality to chain incidents and makes implementation the handleIncident function mandatory for subclasses.
package com.chosengambit.chain;
public abstract class AbstractIncident implements IIncident {
protected AbstractIncident nextHandler;
public enum IncidentType {
MINOR, MAJOR, CRITICAL
}
@Override
public void setNextHandler(AbstractIncident nextHandler) {
this.nextHandler = nextHandler;
}
@Override
public abstract void handleLog(IncidentType type, String message);
}
JavaIncident implementations
We can now implement the three incident types, minor, major and critical.
package com.chosengambit.chain;
public class MinorIncident extends AbstractIncident {
@Override
public void handleIncident(IncidentType type, String message) {
if (type == AbstractIncident.IncidentType.MINOR) {
// create log of incident in database
System.out.println("[Minor incident]: "+message);
}
else {
if (nextHandler != null) {
nextHandler.handleIncident(type, message);
}
}
}
}
Javapackage com.chosengambit.chain;
public class MajorIncident extends AbstractIncident {
@Override
public void handleIncident(IncidentType type, String message) {
if (type == AbstractIncident.IncidentType.MAJOR) {
// log to database
// Send email to administrator
System.out.println("[Major incident]: "+message);
}
else {
if (nextHandler != null) {
nextHandler.handleIncident(type, message);
}
}
}
}
Javapackage com.chosengambit.chain;
public class CriticalIncident extends AbstractIncident {
@Override
public void handleIncident(IncidentType type, String message) {
if (type == AbstractIncident.IncidentType.CRITICAL) {
// log to database
// Send message to supplier
System.out.println("[Critical incident]: "+message);
}
else {
if (nextHandler != null) {
nextHandler.handleIncident(type, message);
}
}
}
}
JavaThe incident manager
The incident manager holds the first element of the chained incident handlers. Maybe the incident classes had better be named with the word “Handler” attached to it, but for now let’s stick with it. We’ll do the chaining in our Main class which ties everything together.
package com.chosengambit.chain;
public class IncidentManager {
private AbstractIncident chain;
public void initChain(AbstractIncident incident) {
this.chain = incident;
}
public void createIncident(AbstractIncident.IncidentType type, String message) {
if (chain != null) {
chain.handleIncident(type, message);
}
}
}
JavaSetting up the chain
The minor incident will have a reference to major incident so it can escalate to it. The major incident will have a critical incident to escalate to. This order can be changed at runtime as you wish. This flexibility is an important aspect of the pattern. The responsible handler will automatically be found in the chain.
package com.chosengambit.designpatterns;
import com.chosengambit.chain.*;
public class Main {
public static void main(String[] args) {
try {
// Create incident handlers
AbstractIncident minor = new MinorIncident();
AbstractIncident major = new MajorIncident();
AbstractIncident critical = new CriticalIncident();
// Chain the handlers, can be done at runtime as well
minor.setNextHandler(major);
major.setNextHandler(critical);
// Invoke incident
IncidentManager manager = new IncidentManager();
manager.initChain(minor);
manager.createIncident(AbstractIncident.IncidentType.CRITICAL, "A critical incident occured!");
manager.createIncident(AbstractIncident.IncidentType.MAJOR, "A major incident occured!");
manager.createIncident(AbstractIncident.IncidentType.MINOR, "A minor incident occured!");
}
catch (Exception e) {
System.out.println(e.toString());
}
}
}
JavaRunning the code
Output:
[Critical incident]: A critical incident occured!
[Major incident]: A major incident occured!
[Minor incident]: A minor incident occured!
C# Example
In the C# (console application) example I renamed some of the classes to include the word Handler this time.
Interface and abstract class
We’ll start with the interface for the IncidentHandler again.
namespace DesignPatterns.Chain
{
internal interface IIncidentHandler
{
public void HandleIncident(AbstractIncidentHandler.IncidentType type, string message);
public void SetNextHandler(IIncidentHandler nextHandler);
}
}
C#The AbstractIncidentHandler implements one of the interface methods while making the other one mandatory to implement for sub classes.
namespace DesignPatterns.Chain
{
abstract internal class AbstractIncidentHandler : IIncidentHandler
{
protected IIncidentHandler? nextHandler;
public enum IncidentType
{
MINOR, MAJOR, CRITICAL
}
public void SetNextHandler(IIncidentHandler nextHandler)
{
this.nextHandler = nextHandler;
}
public abstract void HandleIncident(IncidentType incidentType, string message);
}
}
C#Incident implementations
namespace DesignPatterns.Chain
{
internal class MinorIncidentHandler : AbstractIncidentHandler
{
public override void HandleIncident(IncidentType incidentType, string message)
{
if (incidentType == IncidentType.MINOR)
{
// do stuff
Console.WriteLine($"[Minor incident]: {message}");
}
else if (nextHandler != null)
{
nextHandler.HandleIncident(incidentType, message);
}
}
}
}
C#namespace DesignPatterns.Chain
{
internal class MajorIncidentHandler : AbstractIncidentHandler
{
public override void HandleIncident(IncidentType incidentType, string message)
{
if (incidentType == IncidentType.MAJOR)
{
// do stuff
Console.WriteLine($"[Major incident]: {message}");
}
else if (nextHandler != null)
{
nextHandler.HandleIncident(incidentType, message);
}
}
}
}
C#namespace DesignPatterns.Chain
{
internal class CriticalIncidentHandler : AbstractIncidentHandler
{
public override void HandleIncident(IncidentType incidentType, string message)
{
if (incidentType == IncidentType.CRITICAL)
{
// do stuff
Console.WriteLine($"[Critical incident]: {message}");
}
else if (nextHandler != null)
{
nextHandler.HandleIncident(incidentType, message);
}
}
}
}
C#The incident manager
The IncidentManager class now has an extra function SetupChain that creates the chain in order of the handlers parameter for us.
namespace DesignPatterns.Chain
{
internal class IncidentManager
{
public IIncidentHandler? IncidentChainStart { get; set; }
public void CreateIncident(AbstractIncidentHandler.IncidentType type, string message)
{
if (IncidentChainStart != null)
{
IncidentChainStart.HandleIncident(type, message);
}
}
/// <summary>
/// The first item will be the start of the chain
/// Each next item in the list will be added to the current item
/// </summary>
/// <param name="handlers"></param>
public void SetupChain(List<IIncidentHandler> handlers)
{
for (int i = 0; i < handlers.Count; i++)
{
if (i + 1 <= handlers.Count - 1)
{
handlers[i].SetNextHandler(handlers[i + 1]);
}
}
IncidentChainStart = handlers[0];
}
}
}
C#Setting up the chain
using DesignPatterns.Chain;
class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Running Chain of Responsibility");
// create handlers
List<IIncidentHandler> handlers = new List<IIncidentHandler>()
{
new MinorIncidentHandler(),
new MajorIncidentHandler(),
new CriticalIncidentHandler()
};
IncidentManager incidentManager = new IncidentManager();
incidentManager.SetupChain(handlers);
incidentManager.CreateIncident(AbstractIncidentHandler.IncidentType.MINOR, "A minor incident occured!");
incidentManager.CreateIncident(AbstractIncidentHandler.IncidentType.MAJOR, "A major incident occured!");
incidentManager.CreateIncident(AbstractIncidentHandler.IncidentType.CRITICAL, "A critical incident occured!");
}
}
C#Running the code
Output:
Running Chain of Responsibility
[Minor incident]: A minor incident occured!
[Major incident]: A major incident occured!
[Critical incident]: A critical incident occured!
Conclusion
In the Java and C# examples we created an incident handler chain. The Chain either picks up the type of incident in the handler class based on the incident type or passes it through to the next handler in the chain. A different approach is thinkable, where a handler class both handles and passes the incident through. The exact working and decision making of the chain of responsibility should be as transparent as possible.
Class diagram
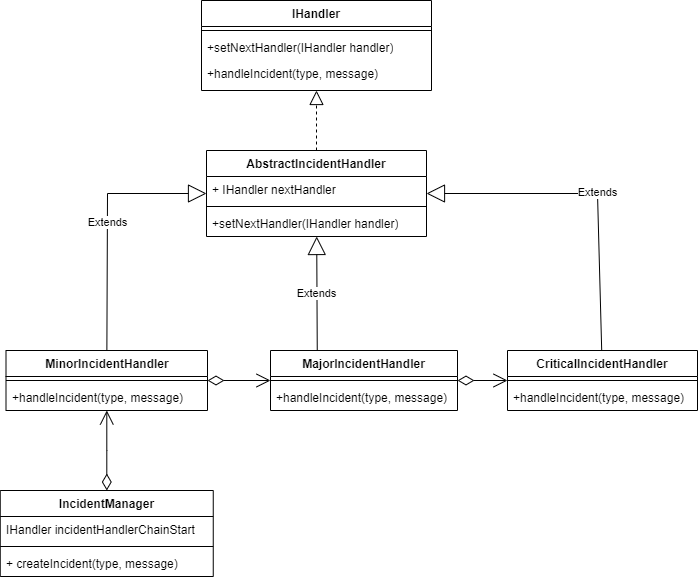
References
Freeman, E., Bates, B., & Sierra, K. (2004). Head first design patterns.
Gamma, E., Helm, R., Johnson, R., & Vlissides, J. (1994). Design Patterns: Elements of Reusable Object-Oriented Software.
Wikipedia contributors. (2024). Software design pattern. Wikipedia. https://en.wikipedia.org/wiki/Software_design_pattern